So we finished the boring parts, in this guide we are going to integrate Paynow using Python
1. Initialize Paynow
Before you can do anything you have to include PayNow and initialize it first
# include paynow here
from paynow import Paynow
paynow = Paynow(
'INTEGRATION_ID',
'INTEGRATION_KEY',
'http://example.com/gateways/paynow/update',
'http://example.com/return?gateway=paynow'
)
This creates a PayNow instance, your keys should be correct or it will raise Integration Exception
on checkout. Errors can be annoying.
2. Creating a payment
The next stage involves adding a payment to the PayNow instance you have created:
import time
invoice_name = "Invoice #100"
user_email = "john@example.co.zw" <em># get email from post request here</em>
payment = paynow.create_payment(invoice_name, user_email)
This adds a payment to your instance you create in step 1. Once payment succeeds PayNow will send an email to the customer for transparence and reference purposes.
If you have one product you can add it like this:
payment.add('Sadza and Beans', 1.25)
If you have more than one products then you can add them like this
products = [Dict1, Dict2, Dict3, ...., Dict10 ]
for product in products:
payment.add(product["name"], product["price"])
If those products have quantities or amounts then it will be something like this
products = [
{"name": "Rice", "price": 3.0, "qty": 2},
{"name": "Potatoes", "price": 10.0, "qty": 1},
{"name": "Knife", "price": 4.0, "qty": 1},
<em># Add more products as needed</em>
]
total = 0
tax = 0.01
for product in products:
item_total = product["price"] * product["qty"]
tax_amount = item_total * tax
item_total += tax_amount
total += item_total
payment.add(product["name"], item_total)
You could add things like coupons, discounts, promotions and what what but well I will leave that you. Code wise you can also use things such as classes but well thats up to you.
Sending payment to PayNow
After adding payment the next stage is sending the payment to payment to PayNow
response = paynow.send_mobile(payment, '077777777', 'ecocash')
Here were requesting for a mobile transaction to occur between our Paynow account and 0777777777 ‘s Ecocash.
The number you get from user through forms or from database. The platform(‘ecocash’) can be retrieved from user through forms or by auto detection
phone = "07777777"
platform = ""
if phone.startswith('071'):
platform= "onemoney"
elif (phone.startswith('073')):
platform = "telecash"
else
platform = "ecocash"
# Then
response = paynow.send_mobile(payment, phone, platform);
Your users will love this ๐๐๐
So this part alone should send a message to your customer mobile asking for payment:
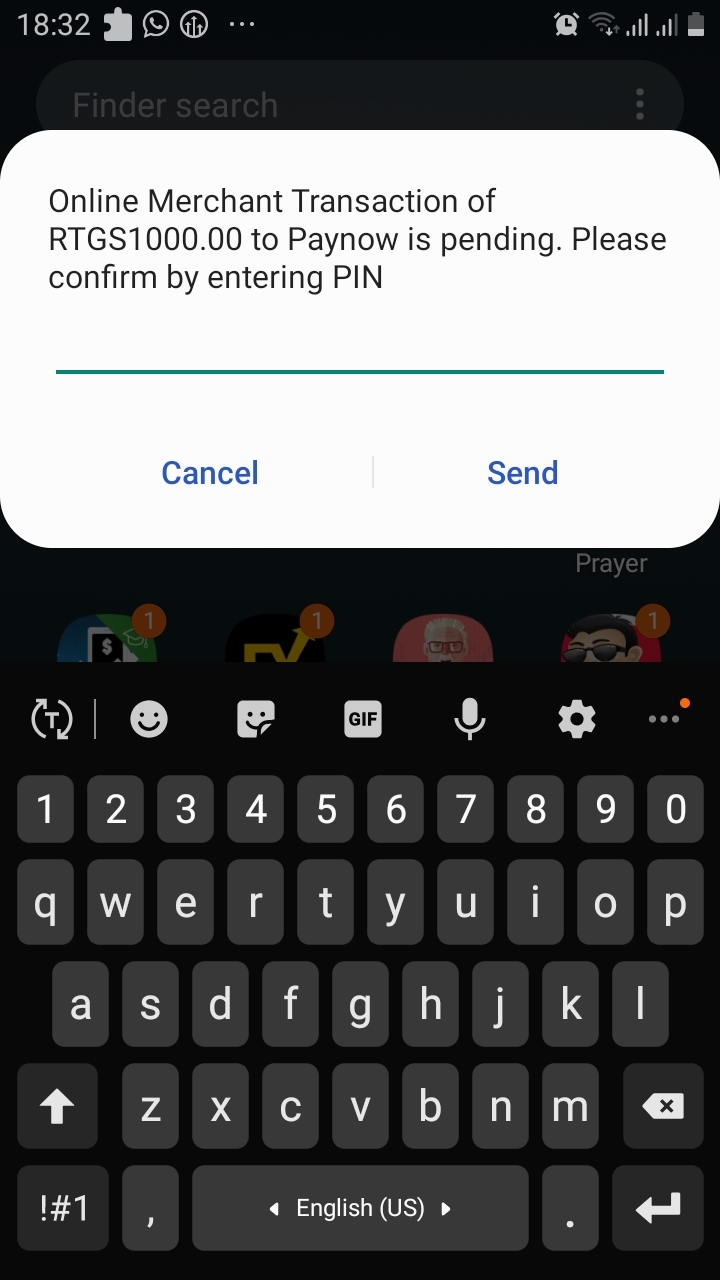
Of course there will be some small differences.
OK that’s it for this one in the next tutorial we will talk about checking payments.
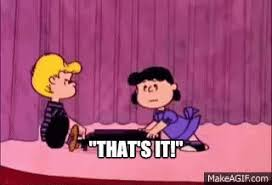
References
Paynow Quickstart guide with Python
Paynow API Reference